C++ is a powerful programming language that is widely used in various domains including system/software development, game development, high-performance applications, embedded systems, and more. Here’s a brief introduction to C++ programming:
- History and Overview:
- C++ was developed by Bjarne Stroustrup at Bell Labs in the early 1980s as an extension of the C programming language.
- It was designed with a focus on efficiency, flexibility, and compatibility with C.
- C++ is an object-oriented programming (OOP) language, which means it supports concepts like classes, objects, inheritance, polymorphism, encapsulation, and abstraction.
- It also supports generic programming features like templates, which allow for the creation of flexible and reusable code.
- Syntax:
- C++ syntax is similar to C, but with additional features for object-oriented programming.
- A C++ program typically starts with the inclusion of header files, followed by a
main()
function which serves as the entry point of the program. - Statements in C++ end with a semicolon
;
. - C++ supports various data types including integers, floating-point numbers, characters, arrays, pointers, and more.
- Control flow statements like
if
,else
,for
,while
,switch
, etc., are used for decision making and looping.
- Object-Oriented Programming (OOP):
- In C++, classes are used to define new data types that bundle data and operations together.
- Objects are instances of classes. They encapsulate data and behavior.
- Inheritance allows a class to inherit properties and behavior from another class.
- Polymorphism enables the use of a single interface for different data types or objects.
- Memory Management:
- C++ provides control over memory management through features like dynamic memory allocation and deallocation using
new
anddelete
operators. - Pointers are extensively used in C++ for memory manipulation and efficient data access.
- C++ provides control over memory management through features like dynamic memory allocation and deallocation using
- Standard Template Library (STL):
- The STL is a powerful library in C++ that provides a set of reusable generic data structures and algorithms.
- It includes containers (like vectors, lists, maps, etc.) and algorithms (like sorting, searching, etc.) that operate on these containers.
- Compile and Link:
- C++ programs are typically compiled into machine code using a compiler like GCC, Clang, or Visual C++ Compiler.
- The compiled code is then linked with libraries to create an executable file.
Overall, C++ is a versatile language known for its performance, flexibility, and wide range of applications. Learning C++ opens up opportunities to work on various software projects and systems programming tasks.
CS107: C++ Programming Exam Quiz Answers
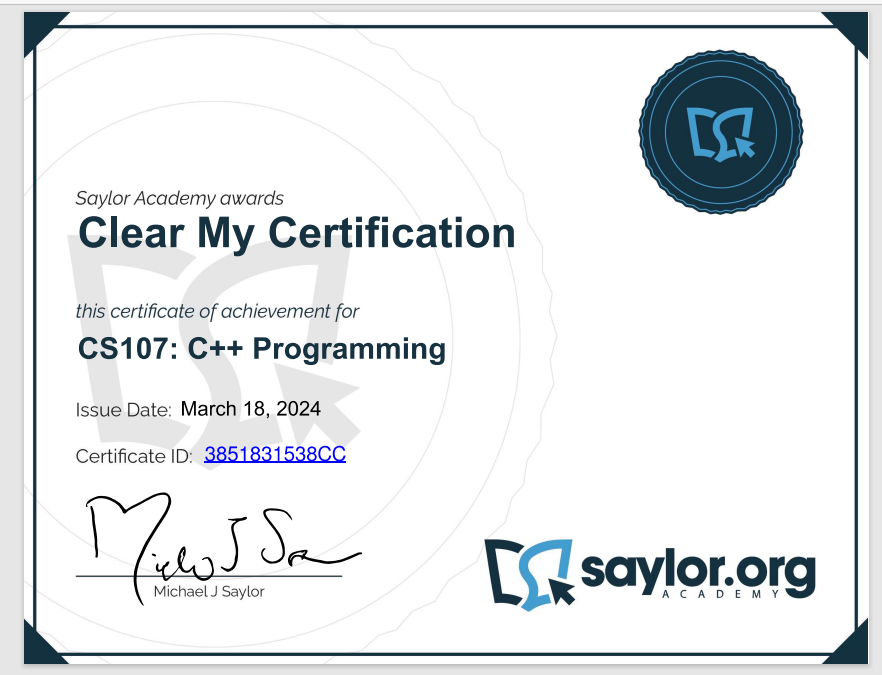
Question 1: The first program ever written was written for which device? Select one:
- Analytical engine
- ENIAC
- Altair
- Von Neumann Architecture
Question 2: To create a program in Eclipse, which of the following must you first create? Select one:
- C++ class
- C++ project
- C++ package
- C++ program file
Question 3: This code produces an error message. Where will the error occur, and why?
1 bool a = 1;
2 int b = 2 + 4;
3 float c = 4;
4 counts<<a + b<<endl;
Select one:
- Line 1: a is a boolean variable, so it cannot accept an integer
- Line 2: Any calculations must be on the left side of the assignment statement
- Line 3: Since c is a float, we must assign it the value 4.0
- Line 4: You must complete the calculations before you can request to output
Question 4: What is the first output on the screen after the following code is compiled and its executable run?
1 #include <iostream>
2 #include <string>
3 #include <sstream>
4 using namespace std;
5 int main () {
6 string str;
7 float price = 0;
8 int quantity = 0;
9 counts << “Enter price: “;
10 getline (cin, str);
11 stringstream (str) >> price;
12 counts << “Enter quantity: “;
13 getline (cin, str);
14 stringstream (str) >> quantity;
15 counts << price * quantity << endl;
16 return 0;
17}
Select one:
- Enter:
- Enter price:
- Enter quantity:
- Enter >>price
Question 5: Which of the following will allow the user to read input to collect the value of the variable age? Select one:
- cin<<age;
- cin>>age;
- count<<age;
- count>>age;
Question 6: Assume that you have two variables, a1 = 5 and a2 = 2, both declared as an int. What is the result of a3 with this calculation?
double a3 = double (a1 % a2)
Select one:
- 1.0
- 1.5
- 2.0
- 2.5
Question 7: Which of the following statements about variable names is true? Select one:
- A valid variable name can be up to 40 characters in length.
- A valid variable name can start with a number and end with a number.
- A valid variable name may consist of letters, digits, and the underscore (_) character.
- A valid variable name may contain uppercase letters, which are no different than lowercase letters.
Question 8: Assume that you have two variables, a1 = 5 and a2 = 2, both declared as an int. What is the result of a3 with this calculation?
int a3 = a1/ a2
Select one:
- 2
- 1.0
- 2.0
- 2.5
Question 9: Given the values a = 5, b = 6, c = 2, and d = 3, what is the value of result for this formula?
int result = a % c + b + d * a
Select one:
- 22
- 50
- 55
- 56
Question 10: Which order will the operations in this formula be calculated in? Use the number beneath each operation sign for reference.
double result = 3 + 5 % 2 – 1 * (3 + (8 – 2) / 3)
1 2 3 4 5 6 7
Select one:
- 1, 2, 3, 4, 5, 6, 7
- 2, 4, 7, 1, 3, 5, 6
- 6, 7, 5, 1, 2, 3, 4
- 6, 7, 5, 2, 4, 1, 3
Question 11: What is the output after the following code is compiled and its executable run?
#include <iostream>
using namespace std;
int main () {
int a, b, c;
a = 5;
b = 6;
c = (a>b)? a: b;
count << c << “\n”;
return 0;
}
Select one:
- 1
- 5
- 6
- 11
Question 12: What type of conditional is executed in this code?
if (x > 0)
{
count << “x is positive” << endl;
} else if (x < 0)
{
count << “x is negative” << endl;
} else
{
count << “x is zero” << endl;
}
Select one:
- Alternative execution
- Chained conditional
- Nested conditional
- Unary conditional
Question 13: While debugging in Eclipse, which key do you use to step over the call? Select one:
- F5
- F6
- F7
- F8
Question 14: C++ provides standard mechanisms to check if the allocation was successful. Which of the following is one way to do this? Select one:
- Use the nothrow method
- Use the noallocate method
- Check the data before it is entered
- Use a bad_allocation_fail exception
Question 15: What is the output after this code is compiled and its executable run?
#include <iostream>
using namespace std;
void pn (int x, int& p, int& n) {
p = x-1;
n = x+1;
}
int main () {
int x = 10, y, z;
pn (x, y, z);
count << y << “, “ << z << “\n”;
return 0;
}
Select one:
- 9, 10
- 9, 11
- 10, 11
- 11, 9
Question 16: Which of the following is required to pass an argument by reference? Select one:
- swap (2,3); Pass the values as literals
- swap (&x, &y); Send the address of the variable as an argument
- &a, &b = swap (x, y); It must be returned to a variable as an address
- void swap (int &x, int &y); Receive the variables in the parameter as an address
Question 17: Suppose I had a phone number stored in a string variable named phone. The value in the phone variable is 289–638–4278. What series of statements would change the area code to be expressed in parentheses, as (289)638–4278? Select one:
- phone = “(” + phone. replace (phone. find (‘0’),1,”) “;
- phone. replace (phone. find (‘-‘), “)”);
- phone. insert (0,”(“);
phone. replace (phone. find (‘-‘),1,”) “);
- phone. replace (0,”(“);
phone. replace (phone. find (‘-‘),1,”) “);
Question 18: Which array has more elements, int i[ 3 ][ 5 ] or int j[ 15 ]? Select one:
- i and j are equal
- i is currently larger than j
- j is currently larger than i
- The size of j is dynamic and will expand as needed so j will be larger than i
Question 19: Which of the following situations would be the best time to use destructors? Select one:
- When the object has created an error
- When we want to create a new object
- When we want to release the memory that the object possesses
- When we want to capture the memory that the object possesses
Question 20: When is a constructor function called? Select one:
- Explicitly, whenever a new object of the constructor class is created
- Automatically, whenever a new object of the constructor is destroyed
- Automatically, whenever a new object of the constructor class is created
- If it is defined in the class and called when a new object of this class is created
Question 21: Which of the following is an example of function overloading? Select one:
- void setInfo (string a) {} void (string b) {}
- void setInfo (int a) {} string setInfo (int a) {}
- void setInfo (int a, int b) {} void setInfo (int b, int a) {}
- void setInfo (int a, int b) {} void setInfo (int a, string b){}
Question 22: What does the keyword this represent? Select one:
- A reference to a memory location of a variable
- A pointer to the function that is being executed
- An object whose member function is being executed
- A pointer to the object whose member function is being executed
Question 23: What are the static data members of a class? Select one:
- Members with one unique value for all the objects of that same class
- Members with one unique value for all the objects of multiple classes
- Members with multiple unique values for all the objects of that same class
- Members with multiple unique values for all the objects of multiple classes
Question 24: Given two classes, Person and Student, how do we define within the Student class that Student is a friend of Person? Select one:
- friend class Person ();
- friend Person;
- class friend Person;
- friend class Person;
Question 25: Which of the following is this image an example of?
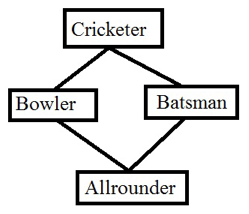
Select one:
- Hierarchical inheritance
- Hybrid inheritance
- Multilevel inheritance
- Multiple inheritance
Question 26: What error is included in this code?
#include <iostream>
#include <math>
using namespace std;
int main ()
{
countdown (3);
return 0;
}
void printLogarithm (double x)
{
if (x <= 0.0) {
count << “Positive numbers only, please.” << endl;
return;
}
double result = log (x);
count << “The log of x is “ << result;
}
void countdown (int n) {
if (n == 0) {
count << “Blastoff!” << endl;
} else {
count << n << endl;
countdown (n-1);
}
}
Select one:
- The functions appear after the main
- Main does not call the correct function
- The functions should have a return value
- The printLogarithm function should contain an else clause
Question 27: Which of the following best describes polymorphism? Select one:
- It uses a derived class in a similar manner as the base class, with the use of pointers
- It takes advantage of the fact that a pointer to a derived class is type-compatible with a pointer to its base class
- It takes advantage of the fact that a class has only a single function with the same data type pointing to the derived class
- It makes sure that all pointers in the derived class point to functions within the base class, but not to the operator members
Question 28: What is the output after this code is compiled and its executable run?
#include <iostream>
using namespace std;
class polygon {
protected:
int width, height;
public:
void setValues (int a, int b) {width=a; height=b;}
};
class output {
public:
void output (int i);
};
void output: output (int i) {
count << i;
}
class rectangle: public polygon, public output {
public:
int area () {return (width * height);}
};
class triangle: public polygon, public output {
public:
int area () {return (width * height / 2);}
};
int main () {
rectangle rct;
triangle trgl;
rct. setValues (4,5);
trgl. setValues (4,5);
rct. output (rct. area ());
count << ” “;
trgl. output (trgl. area ());
count << “\n”;
return 0;
}
Select one:
- 4 5
- 5 4
- 10 20
- 20 10
Question 29: How do we define a mutator named setName? Select one:
- string setName () {return name;}
- void setName () {name = myname;}
- string setName(myname) {name = myname;}
- void setName (string myname) {name = myname;}
Question 30: Which of the following is the correct use of a template? Select one:
- template<typename T>
class Foo {
public:
Foo ();
void someMethod (typename T x);
private:
typename T x;
};
- class Foo {
public:
Foo ();
void someMethod (T x);
private:
T x;
};
- template<typename T>
class Foo {
public:
Foo ();
void someMethod (T x);
private:
T x;
};
- <typename T>template
class Foo {
public:
Foo ();
void someMethod (T x);
private:
T x;
};
Question 31: What import statement is used to read and write from/to files? Select one:
- #include <iostream>
- #include <ofstream>
- #include <ifstream>
- #include <fstream>
Question 32: Given the code below, you want the method to issue an error if b is equal to zero, so that you are not dividing by zero. Write the code in line 3 that issues this error.
1 double division (int a, int b) {
2 if (b == 0) {
3 <Enter code here>
4}
5 returns (a/b);
Select one:
- throws “Division by zero condition!”;
- throw “Division by zero condition!”;
- exception “Division by zero condition!”;
- catch “Division by zero condition!”;
Question 33: Who developed the C++ programming language? Select one:
- Brian Kernighan
- Bjarne Stroustrup
- Dennis Ritchie
- Ken Thompson
Question 34: Why would the output of 13/5 be 2 and not 2.6? Select one:
- When we divide, the result is always a float.
- When we divide, the result is always an integer.
- When we divide two integers, the result will always be an integer.
- When we divide two numbers, the result will be a float, which then gets converted to an integer.
Question 35: Which of the following will allow the user to read input to collect the value of the variable age? Select one:
- cin<<age;
- cin>>age;
- cout<<age;
- cout>>age;
Question 36: Which of the following statements about variable names is true? Select one:
- A valid variable name can be up to 40 characters in length.
- A valid variable name can start with a number and end with a number.
- A valid variable name may consist of letters, digits, and the underscore (_) character.
- A valid variable name may contain uppercase letters, which are no different than lowercase letters.
Question 37: If b = 3 and then a = b++, what do a and b contain? Select one:
- a contains 3, b contains 3
- a contains 3, b contains 4
- a contains 4, b contains 3
- a contains 4, b contains 4
Question 38: When a value is passed to a function, what is the term for the value that is passed? Select one:
- Argument
- Call
- Instance
- Parameter
Question 39: Which of the following statements would produce a True result given this code?
char s1[] = “alpha”;
char s2[] = “alpha”;
char s3[] = “beta”;
char s4[] = “gamma”;
Select one:
- strcmp (s1, s2);
- strcmp (s1, s3);
- strcmp (s3, s4);
- strcmp (s1, “Alpha”);
Question 40: What is the output after this code is compiled and its executable run?
#include <iostream>
#include <string>
using namespace std;
main () {
string B (“money”);
string S (“goods”);
count << “before, B has “ << B;
count << ” and S has “ << S << “; “;
swap (B, S);
count << ” after, B has “ << B;
count << ” and S has “ << S << endl;
return 0;
}
Select one:
- B has goods and S has money
- B has money and S has goods; B has goods and S has money
- after, B has goods and S has money; before, B has money and S has goods
- before, B has money and S has goods; after, B has goods and S has money
Question 41: What is a default constructor in a class definition? Select one:
- Constructor with no functions
- Constructor with no arguments
- Constructor with one argument
- Constructor with name argument
Question 42: When do you use this keyword in a constructor? Select one:
- When there are no parameters
- When the parameters are undefined
- When there are no data members defined
- When the data members have the same name as the parameters passed in
Question 43: In order to use two classes with the same name in C++, you must do which of the following? Select one:
- Use dot notation
- Put it in a separate folder
- Create a different namespace
- Create a different folder and use dot notation
Question 44: What does a derived class inherit from the base class? Select one:
- It inherits every member of a base class
- It only inherits the operator members, not the methods, including constructors and destructors
- It inherits every member of a base class including the operator members but does not include the constructor and destructor
- It inherits every member of a base class but it does not inherit its constructor and destructor, operator members, or its friends
Question 45: Which of the following is a characteristic of an accessor? Select one:
- It requires one argument
- It may accept multiple parameters
- The name of the accessor begins with the Set prefix
- It has a return type consistent with the data being retrieved
Question 46: Which of the following was the first high-level language developed? Select one:
- C
- COBOL
- Fortran
- Java
Question 47: Which object in C++ has access to the standard input of a program (text on the screen)? Select one:
- cin<<
- cin>>
- cout<<
- cout>>
Question 48: Which object in C++ has access to the standard output of a program (the screen)? Select one:
- cin<<
- cin>>
- cout<<
- cout>>
Question 49: Which data type in the C++ language was not included in the C language? Select one:
- boolean
- char
- double
- float
Question 50: What is the value of a in a = 5 + 7 % 2 *3? Select one:
- 2
- 6
- 8
- 12
Question 51: What is the result of this statement, following the correct order of operations?
result = 4 * 2 + 1 * 3 – (2 * 3 + (4 / 2) + 3)
Select one:
- 0
- 9
- 11
- 16
Question 52: What does the statement a = (b = 5, b+1) do? Select one:
- It assigns the value 5 to a and then assigns b+1 to variable a
- It assigns the value 5 to b and then assigns b+1 to variable b
- It assigns the value 5 to a and then assigns b+1 to variable b
- It assigns the value 5 to b and then assigns b+1 to variable a
Question 53: What is the final output of the following code?
int a = 5;
int b = 10;
int c = 3;
for (int x =0; x< 5; x++)
{
a += 2;
b -= 1;
c *= 2;
}
count<<“A is “<<a<<” and C is “<<c<<endl;
Select one:
- A is 10 and C is 30
- A is 10 and C is 96
- A is 15 and C is 30
- A is 15 and C is 96
Question 54: What is a reference to a variable in C++? Select one:
- The size of a variable in computer memory
- The type of a variable in computer memory
- The address that locates a variable in computer memory
- The address that locates the start of a program in computer memory
Question 55: What is the output after this code is compiled and its executable run?
#include <iostream>
#include <string>
using namespace std;
int main () {
string str (“Let us do something crazy”);
cout << “size: ” << str.size() << “, “;
cout << “length: ” << str.length() << “, “;
cout << “capacity: ” << str.capacity() << “, “;
cout << “max size: ” << str.max_size() << “\n”;
return 0;
}
Select one:
- size: 2, length: 2, capacity: 2, max size: 25
- size: 5, length: 5, capacity: 5, max size: 4611686018427387897
- size: 25, length: 5, capacity: 25, max size: 25
- size: 25, length: 25, capacity: 25, max size: 4611686018427387897
Question 56: What will be the output of this program?
1 #include <iostream>
2 using namespace std;
3 int array1[] = {1000, 100, 2100, 1200, 1500};
4 int array2[] = {10, 12, 14, 16, 18};
5 int temp, result = 0;
6 int main ()
7 {
8 for (temp = 0; temp < 5; temp++)
9 {
10 results += array1[temp];
11}
12 for (temp = 0; temp < 4; temp++)
13 {
14 results += array2[temp];
15}
16 counts << result;
17 return 0;
18}
Select one:
- 70
- 5900
- 5952
- 5970
Question 57: Which of the following is the correct definition of a destructor for the class Person? Select one:
- ~Person ();
- delete Person ();
- destroy Person ();
- ~Person (int x, int y);
Question 58: Which line from this code indicates that class Car inherits from class Vehicle?
1 class Vehicle {
2 protected:
3 string licenses;
4 int year;
5 public:
6 Vehicle (const string &myLicense, cons tint myYear): license(myLicense),
7 years(myYear) {}
8 const string getDesc () const (return license + ” from ” + stringify(year);}
9 const string &getLicense () const {return license;}
10 const int getYear () const {return year;}
11};
12 class Car: public Vehicle {
13 privates: string style;
14 public:
15 Car (const string &myLicense, cons tint myYear, const string &myStyle)
16 Vehicle (myLicense, myYear), style(myStyle) {}
17 const string &getStyle () {return style;}
18 const string getDesc () {return stringify(year) + ‘ ‘ + style +: ” + license;}
Select one:
- 1
- 6
- 12
- 15
Question 59: What is the best definition of class? Select one:
- It is a group of elements of the same type
- It is a group of functions of different types
- It is an expanded concept of a data structure that can hold data
- It is an expanded concept of a data structure that can hold data and functions
Question 60: What is the output after this code is compiled and its executable run?
#include <iostream>
using namespace std;
class poly {
protected:
int width, height;
public:
void setValues (int a, int b) {width=a; height=b;}
};
class rectangl: public poly {
public:
int area () {return (width * height);}
};
class triang: public poly {
public:
int area () {return (width * height / 2);}
};
int main () {
rectangl r;
triang t;
r. setValues (2,9);
t. setValues (7,8);
cout << r. area () << “, “;
cout << t. area () << endl;
return 0;
}
Select one:
- 2, 7
- 7, 8
- 18, 9
- 18, 28
Question 61: What is the output beginning in line 19 after this code is compiled and its executable run?
1 #include <iostream>
2 #include <string>
3 #include <sstream>
4 using namespace std;
5 struct movies_t {
6 string title;
7 int year;
8 } mn, ys;
9 void pm (movies_t movie);
10 int main () {
11 string ms;
12 mn.title = “2001 A Space Odyssey”;
13 mn.year = 1968;
14 counts << “Enter title: “;
15 getline (cin, ys. title);
16 counts << “Enter year: “;
17 getline (cin, ms);
18 stringstream (ms) >> ys. year;
19 counts << “My favorite movie is: “;
20 pm (mn);
21 counts << “And yours is:\n “;
22 pm (ys);
23 return 0;
24 }
25 void pm (movies_t movie) {
26 counts << movie. title;
27 counts << ” (“ << movie. year << “) \n”;
28 }
Select one:
- 2001 A Space Odyssey
- 2001 A Space Odyssey (1968)
- My favorite movie is: 2001 A Space Odyssey 1968
- My favorite movie is: 2001 A Space Odyssey (1968)
Question 62: What folder is created when you create a project in Eclipse? Select one:
- Class folder
- Project folder
- Default folder
- Application folder
Question 63: What would the output of the following code be?
1 #include <iostream>
2 using namespace std;
3 int a = 10;
4 int b = 5;
5 string c = “pumpkin bread”;
6 string d = “candy apples”;
7 counts<<c<<“is $”<<a<<” and “<<d<<” is $”<<b<<endl;
Select one:
- candy apples are $10 and pumpkin bread is $5
- pumpkin bread is 10 and candy apples is 5
- pumpkin bread is $5 and candy apples are $10
- pumpkin bread is $10 and candy applies is $5
Question 64: Assume that you have two variables, a1 = 5 and a2 = 2, both declared as an int. What is the result of a3 with this calculation?
double a3 = double (a1 / a2)
Select one:
- 1
- 2
- 2.0
- 2.5
Question 65: Given the input of 40000 s, 30000 s, and 70000 m, what data would be needed to ensure thorough coverage analysis?
cin >> income;
cin >> marital_status;
if (marital_status == “s”) // Condition 1
{
if (income <= 30000) // Condition 2
tax = 0.10 * income; // Branch 1
else
tax = 3000 + 0.25 * (income – 30000); // Branch 2
}
else
{
if (income <= 60000) // Condition 3
tax = 0.10 * income; // Branch 3
else
tax = 6000 + 0.25 * (income – 60000); // Branch 4
}
Select one:
- 60000 m
- 65000 m
- 20000 s
- 50000 s
Question 66: When is it possible in C++ to have two different functions with the same name? Select one:
- When their parameter types are different
- When the parameters and the parameter types are the same
- When the parameter types or number of parameters are different
- When the parameter types are the same but the parameters are different
Question 67: What is the output after this code is compiled and its executable run?
#include <iostream>
#include <string>
using namespace std;
int main () {
string S1;
string S2=”Writing “;
string S3=”print 10 and then 5 more”;
S1. append(S2);
S1. append(S3,6,3);
S1. append (“dots are cool”,5);
S1. append (“here: “);
S1. append (10, ‘.’);
S1. append (S3, 8, 11);
S1. append (” “);
S1. append (S3,20, S3. size ()-1);
count << S1 << endl;
return 0;
}
Select one:
- Writing dots here: ………. and then more
- Writing 5 dots here: …… and then 10 more……….
- Writing 10 dots here: ………. and then 5 more
- Writing 10 dots here: ………. and then 10 more……….
Question 68: Which of the following is the correct format to declare a union? Select one:
- union employee {
int age;
long salary;
};
- union Employee {
int age;
long salary;
} employee;
- union employee {
int age;
long salary;
} Employee;
- union Employee {
int age;
long salary;
};
Question 69: What is special about the union data type? Select one:
- It is named differently than other data types
- It can hold more information than other data types
- It allows you to group multiple like items together into a single set
- It allows the same portion of memory to be accessed as different data types
Question 70: Consider these two classes and the main method. The compiler generates an error message on line 35. What is the error?
1 #include <iostream>
2 using namespace std;
3 #include <string>
4
5 class Square;
6
7 class Rectangle {
8 int width, height;
9 public:
10 int area ()
11 {return (width * height);}
12 void converts (Square a);
13};
14
15 class Square {
16 friend class Rectangle;
17 privates:
18 int side;
19 public:
20 Square (int a): side(a) {}
21 int perimeter (Square a);
22};
23 void Rectangle: convert (Square a) {
24 widths = a. side;
25 heights = a. side;
26}
27 int Square: perimeter (Square a)
28 {
29 return a. side + a. side + a. side + a. side;
30}
31 int main () {
32 Rectangle rect;
33 Square sqr (4);
34 rect. convert(sqr);
35 int perimeter = rect. perimeter(sqr);
36 cout <<perimeter<<endl;
37 return 0;
38}
Select one:
- perimeter does not return a value
- square does not have access to perimeter
- rectangle does not have access to perimeter
- We need to pass a rectangle to perimeter, not a square
Question 71: Given this code, which line will provide an error?
1 #include <iostream>
2 using namespace std;
3 class Box {
4 public:
5 double lengths;
6 void setWidth (double wid);
7 double getWidth (void);
8
9 privates:
10 double widths;
11};
12
13 double Box: getWidth(void) {
14 return width;
15}
16 void Box: setWidth (double wid) {
17 widths = wid;
18}
19 int main () {
20 Box box;
21 boxes. length = 10.0;
22 count << “Length of box: ” << box. length <<endl;
23 boxes. width = 10.0;
24 boxes. setWidth (10.0);
25 count << “Width of box: ” << box. getWidth () <<endl;
26 return 0;
27}
Select one:
- Line 21
- Line 22
- Line 23
- Line 24
Question 72: Which of the following offers us mechanisms to perform inheritance relationships that produce different behaviors for the same function call or operator, but perform the necessary lookup at compile-time rather than run-time? Select one:
- Templates
- Inheritance
- Abstract functions
- Function overloading
Question 73: When opening a file for output, which optional mode parameter deletes any previous content before replacing with the new content? Select one:
- ios: app
- ios: ate
- ios: trunc
- ios: overwrite
Question 74: Given this code, which line would produce an error?
1 try
2 {quotient = divide (num1, num2);}
3 cout << “The quotient is ” << quotient << endl;
4 catches (string exceptionString)
5 {cout << exceptionString;}
Select one:
- Line 2
- Line 3
- Line 4
- Line 5
Question 75: When writing code using Eclipse, once you have added a project, you need to add a file with code. From project explorer, where do you create a page to write your code? Select one:
- New → Add Code
- New → Source File
- Project Type → Empty Project
- Select Configuration → Source File
Question 76: Assume that you have two variables a1 and a2. a1 is declared as an int, and a2 is declared as a double. Which of the following would be a correct assignment statement? Select one:
- a1= a2;
- a1 = int(a2);
- a1 = double(a2);
- double a1 = a2;
Question 77: What does the & operator do? Select one:
- It uses OR operation bit by bit between two variables
- It represents the OR comparison between two variables
- It uses AND operation bit by bit between two variables
- It represents the AND comparison between two variables
Question 78: What is the output after the following code is compiled and its executable run?
#include <iostream>
using namespace std;
int main () {
for (int n = 10; n>0; n–) {
cout << n << “, “;
if (n == 3 ){
cout << “countdown ended!”;
break;
}
}
return 0;
}
Select one:
- 10, 9, 8, 7, 6, 5, 4, countdown ended!
- 10, 9, 8, 7, 6, 5, 4, 3 countdown ended!
- 10, 9, 8, 7, 6, 5, 4, 3, countdown ended!
- 10, 9, 8, 7, 6, 5, 4, 3, 2 countdown ended!
Question 79: Using the debugger in Eclipse, what does the Detail Formatter do? Select one:
- Creates a breakpoint on a specific field
- Allows you to delete and deactivate stop points
- Use Java code to define how a variable is displayed
- Uses the toString () method to determine how to display the variable
Question 80: Which branch in this code is tested by the input 40000 s?
cin >> income;
cin >> marital_status;
if (marital_status == “s”) // Condition 1
{
if (income <= 30000) // Condition 2
tax = 0.10 * income; // Branch 1
else
tax = 3000 + 0.25 * (income – 30000); // Branch 2
}
else
{
if (income <= 60000) // Condition 3
tax = 0.10 * income; // Branch 3
else
tax = 6000 + 0.25 * (income – 60000); // Branch 4
}
Select one:
- Branch 1
- Branch 2
- Branch 3
- Branch 4
Question 81: What is the output after this code is compiled and its executable run?
#include <iostream>
using namespace std;
int d (int a, int b = 4) {
int r;
r = a / b;
return r;
}
int main () {
cout << d (16) << “, ” << d (24, 6) << “\n”;
return 0;
}
Select one:
- 4, 4
- 4, 6
- 6, 24
- 24, 6
Question 82: What might be a good reason to write code in functions instead of part of the main function? Select one:
- Programs don’t run well unless they are inside functions.
- This allows the programmer to get creative with the code.
- This makes the program smaller by eliminating repetitive code.
- This moves the focus of the code to the method instead of the main.
Question 83: What is the final output of this code?
double tmpC [5] = {-10.0, -8.0, -6, -4.0, -2.0};
fprintf (“%8s %8s\n”, “Celsius”, “Fahrenheit”);
for (i=0; i<3; i++) {
tmpF = ((tmpC[i] * (9.0/5.0)) + 32.0);
fprintf (“%10.2f %10.2f\n”, tmpC[i], tmpF);
}
Select one:
- Celsius Fahrenheit
-10 23.8
-8 25.8
-6 27.8
-4 29.8
-2 31.8
- Fahrenheit Celsius
-10 23.8
-8 25.8
-6 27.8
-4 29.8
-2 31.8
- Celsius Fahrenheit
-10.00 23.80
-8.00 25.80
-6.00 27.80
- Celsius Fahrenheit
-10.00 23.80
-8.00 25.80
-6.00 27.80
-4.00 29.80
-2.00 31.80
Question 84: What is the output after this code is compiled and its executable run?
#include <iostream>
#include <string>
using namespace std;
main () {
string F (“com”);
string S (“ilovecplusplus”);
string C (“http://”);
string H;
string url;
H = “www.” + S + ‘.’ + F;
url = C + H;
cout << url << endl;
return 0;
}
Select one:
- ilovecplusplus.com
- www.ilovecplusplus.com
- http://ilovecplusplus.com
- http://www.ilovecplusplus.com
Question 85: Given this code, which of the following would you use to loop through all of the elements?
int values [20];
Select one:
- for (int i = 0; i<20; ++i) {}
- for (int i = 0; i<=20; ++i) {}
- for (int i = 0; i<values. size (); ++i) {}
- for (int i = 0; i<=values. size (); ++i) {}
Question 86: Which of the following is the correct definition of a default constructor for the class Person? Select one:
- Person (name, id)
{
name = name;
id = id;
}
- Person ()
{
name = “”;
id = “”;
}
- Person (string name, string id)
{
name = “”;
id = “”;
}
- Person
{
name = “”;
id = “”;
}
Question 87: Which of the following statements for overloaded operators is true? Select one:
- It is possible to change the precedence of operators
- New operators such as **, <> or &/ can be created
- The overloads of operators && and || lose their short-circuit evaluation
- &&, ||, and, (comma) maintain their special sequencing properties when overloaded
Question 88: What is the correct format to use the keyword this for setting the data member name? Select one:
- name = this.name;
- this.name = name;
- name = this->name;
- this->name = name;
Question 89: Which statement is true regarding exception handling? Select one:
- An exception is caught in a try block
- An exception can be thrown in a try block
- A try block can be followed by only one catch block
- It combines the normal case code with the code that handles exceptions